
Vue Js Year picker Example:In Vue.js, you can create aVue Js year picker component by using a combination of HTML, CSS, and JavaScript. One way to implement it is by creating a dropdown select menu that contains a range of years.
You can populate the dropdown menu dynamically by using a loop in Vue’s template syntax, iterating over the desired year range. You can then use Vue’s two-way data binding to store the selected year value in the component’s data.
Whenever the user selects a year from the dropdown, the value will be updated in the component’s data, allowing you to use it as needed in your application.
How can I implement Vue Js Year Picker ?
The code provided is an example of a Vue js Year Picker. It uses the Vue.js framework to create a simple Vue Js year Picker interface.
In the HTML section, there is a <select>
element that binds to the selectedYear
data property using v-model
. This property represents the currently selected year. Inside the <select>
, there is an initial disabled and selected option prompting the user to select a year.
In the JavaScript section, a Vue app is created using Vue.createApp()
. It defines the data properties: selectedYear
, which holds the selected year, startYear
, which represents the starting year for the year list, and endYear
, which is set to the current year.
The app also defines a computed property called yearList
. This property generates an array of years, starting from the endYear
and going backwards until the startYear
. The v-for
directive is used to loop over this array and dynamically generate <option>
elements with the corresponding year as their values.
Overall, this code sets up a basic Vue js year Picker component that allows the user to select a year from a dropdown list.
Vue Js Year Picker Example
<div id="app">
<h3>Vue Js Year Picker Example</h3>
<select v-model="selectedYear">
<option value="" disabled selected>Select a year</option>
<option v-for="year in yearList" :value="year">{{ year }}</option>
</select>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
selectedYear: '',
startYear: 1990,
endYear: new Date().getFullYear(),
}
},
computed: {
yearList() {
const years = [];
for (let i = this.endYear; i >= this.startYear; i--) {
years.push(i);
}
return years;
},
},
});
app.mount('#app');
</script>
Output of Vue Js Year Picker Example
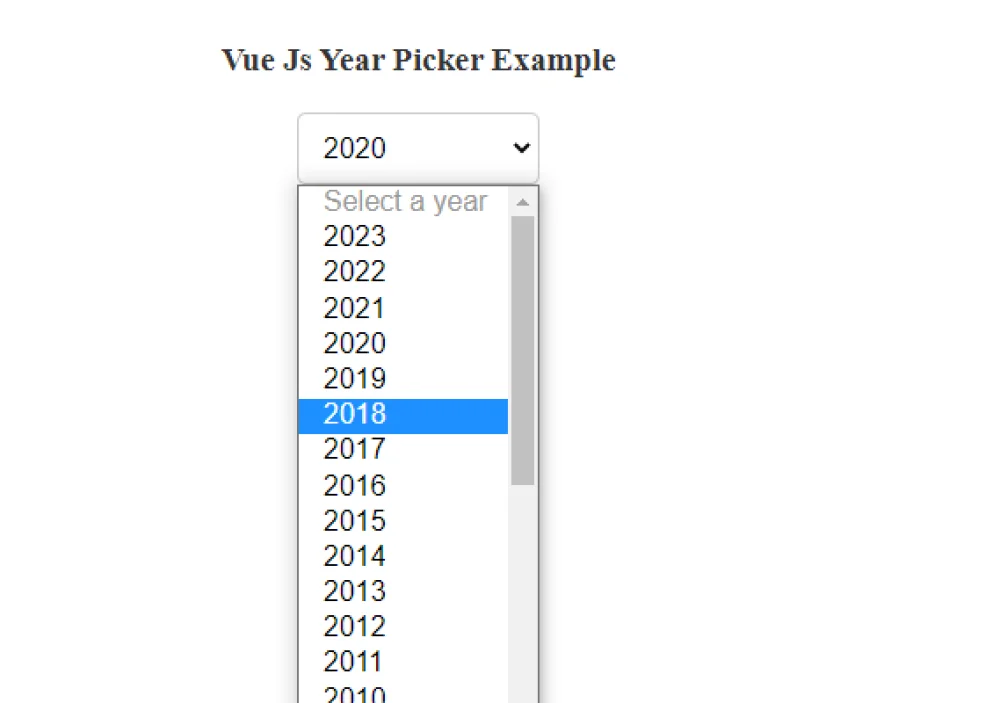
Vue js Year Picker with CSS Code Demo
The Vue js Year Picker is a user interface component that allows users to select a year from a predefined range. It can be implemented using Vue.js and styled using CSS. A code demo showcasing this functionality can be found at [Demo].
Vue Js Year Picker – Css Code
#app {
text-align: center;
margin-top: 50px;
}
h3 {
color: #333;
}
select {
padding: 10px;
font-size: 16px;
border-radius: 5px;
border: 1px solid #ccc;
}
option[disabled] {
color: #999;
}
option[selected] {
font-weight: bold;
}